Professional Python
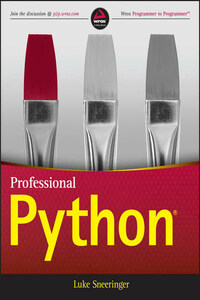
Master the secret tools every Python programmer needs to know
Professional Python goes beyond the basics to teach beginner- and intermediate-level Python programmers the little-known tools and constructs that build concise, maintainable code. Design better architecture and write easy-to-understand code using highly adoptable techniques that result in more robust and efficient applications. Coverage includes Decorators, Context Managers, Magic Methods, Class Factories, Metaclasses, Regular Expressions, and more, including advanced methods for unit testing using asyncio and CLI tools. Each topic includes an explanation of the concept and a discussion on applications, followed by hands-on tutorials based on real-world scenarios. The "Python 3 first" approach covers multiple current versions, while ensuring long-term relevance.
Python offers many tools and techniques for writing better code, but often confusing documentation leaves many programmers in the dark about how to use them. This book shines a light on these incredibly useful methods, giving you clear guidance toward building stronger applications.
Learn advanced Python functions, classes, and libraries
Utilize better development and testing tools
Understand the "what," "when," "why," and "how"
More than just theory or a recipe-style walk-through, this guide helps you learn – and understand – these little-known tools and techniques. You'll streamline your workflow while improving the quality of your output, producing more robust applications with cleaner code and stronger architecture. If you're ready to take your Python skills to the next level, Professional Python is the invaluable guide that will get you there.